Flutter is a framework which is specially expertise on different kinds of animations compared to native languages. We can use a lot of animations which are absolutely free out there. This article will show you the best way of how to use flutter animations which are most commonly used.
There are a lot of animations which we can get from “pub.dev”, but flutter SDK also provides some implicit animations built-in. Let’s discuss some of the animations.
Intro Views
This is a dependency that we can create some of the beautiful intro sliders when the app launches for the first time. interviews are specially designed and inspired by Paper Onboarding from scratch.
It has features like easy addition of pages, Circular page reveals, Cool animations, Skip button for skipping app intro and also custom font selection. It does have Material design perfectly.
We need to add dependency to access the features of it
intro_views_flutter: '^2.8.1'
Create a page using Page View model class in this dependency.
Final page = new PageViewModel( pageColor: const Color(0xFF607D8B), iconImageAssetPath: 'assets/taxi-driver.png', iconColor: null, bubbleBackgroundColor: null, body: Text( 'Easy cab booking at your doorstep with cashless payment system', ), title: Text('Cabs'), mainImage: Image.asset( 'assets/taxi.png', height: 285.0, width: 285.0, alignment: Alignment.center, ), titleTextStyle: TextStyle(fontFamily: 'MyFont', color: Colors.white), bodyTextStyle: TextStyle(fontFamily: 'MyFont', color: Colors.white), );
You can create a number of Page View Models. It depends on how many screens we need on the intro slider. Add this to IntroViewsFlutter in order to get all the pages listed. You will be seeing listeners and a lot more attributes to style this according to you.
final Widget introViews = new IntroViewsFlutter( [page], onTapDoneButton: (){ //Void Callback }, showSkipButton: true, pageButtonTextStyles: new TextStyle( color: Colors.white, fontSize: 18.0, fontFamily: "Regular", ), );
Slider Button
This is used for both iOS and Android and even on the Web. This package provides an easy implementation of a Slider Button. It is a highly customisable iphone alike looking widget.
Add the below dependency in “pubspec.yaml” file to get the all packages used in this animation.
slider_button: ^0.5.0
Add the package below to get access to it.
import 'package:slider_button/slider_button.dart';
Type 1(Circular)
Center(child: SliderButton( action: () { ///Do something here Navigator.of(context).pop(); }, label: Text( "Slide to cancel Event", style: TextStyle( color: Color(0xff4a4a4a), fontWeight: FontWeight.w500, fontSize: 17), ), icon: Text( "x", style: TextStyle( color: Colors.white, fontWeight: FontWeight.w400, fontSize: 44, ), ),
Type 2(Rectangular)
SliderButton( action: () { ///Do something here OnSlide }, ///Put label over here label: Text( "Slide to cancel !", style: TextStyle( color: Color(0xff4a4a4a), fontWeight: FontWeight.w500, fontSize: 17), ), icon: Center( child: Icon( Icons.power_settings_new, color: Colors.white, size: 40.0, semanticLabel: 'Text to announce in accessibility modes', )), ///Change All the color and size from here. width: 230, radius: 10, buttonColor: Color(0xffd60000), backgroundColor: Color(0xff534bae), highlightedColor: Colors.white, baseColor: Colors.red, );
There are some more usages which are used here. You can refer to the “Pub.dev” page to get more information.
Rating Bar
Rating Bars are usually common in every programming language. Coming to flutter, It provides you the immense feeling of different icons to add in the rating bar. It has various attributes in this dependency in order to make the rating bar more stylier according to your usage. A simple but fully customizable rating bar in flutter.
- We can set minimum and maximum rating
- Any widgets can be used as a rating or indicator items
- Supports vertical layout
- Glows or gives shine on user interaction with the widget
- Supports RTL model.
Add the below code to get the rating bar in your project.
RatingBar( initialRating: 3, minRating: 1, direction: Axis.horizontal, allowHalfRating: true, itemCount: 5, itemPadding: EdgeInsets.symmetric(horizontal: 4.0), itemBuilder: (context, _) => Icon( Icons.star, color: Colors.amber, ), onRatingUpdate: (rating) { print(rating); }, );
If you want to use your own icons then use it like this
RatingBar( initialRating: 3, direction: Axis.horizontal, allowHalfRating: true, itemCount: 5, ratingWidget: RatingWidget( full: _image('assets/heart.png'), half: _image('assets/heart_half.png'), empty: _image('assets/heart_border.png'), ), itemPadding: EdgeInsets.symmetric(horizontal: 4.0), onRatingUpdate: (rating) { print(rating); }, );
Toggle Switch
Toggle switch is something which we can select and set our desired option. In flutter it is fully customized with desired icons,width,texts,colors,corner radius etc.
For getting started, we need to add the following dependency into our “pubspec.yaml” file under the dependencies.
toggle_switch: "^0.1.4"
Add the following package which comes from dependency to access Toggle Switch features.
import 'package:toggle_switch/toggle_switch.dart';
Usage example:
ToggleSwitch( minWidth: 90.0, cornerRadius: 20, activeBgColor: Colors.green, activeTextColor: Colors.white, inactiveBgColor: Colors.grey, inactiveTextColor: Colors.white, labels: ['YES', 'NO'], icons: [FontAwesomeIcons.check, FontAwesomeIcons.times], onToggle: (index) { print('switched to: $index'); })
Simple Animations
These are simple animations where we can create them easily using these features. It supports in all the formats including Web, iOS & Android.
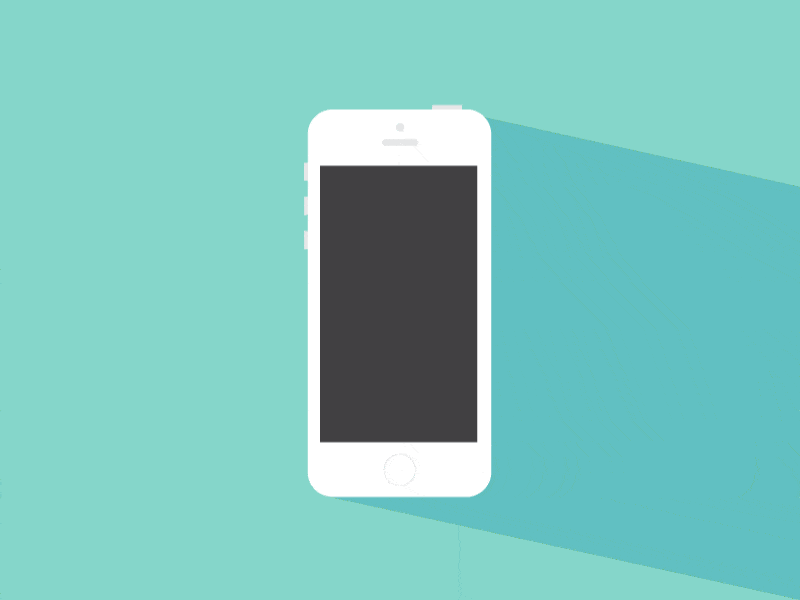
- Easily we can create custom animations in Stateless widget.
- Any widgets can be used as a rating or indicator items
- We can animate Multiple properties at once.
- Designed with type-safety in mind.
- Simplified working with AnimationController instances.
Add the following dependency
simple_animations: ^2.2.1
Add the following package
import 'package:simple_animations/simple_animations.dart';
There are three types of animations comes under simple features
1.) Stateless Animations
PlayAnimation( // <-- specify type of animated variable tween: Colors.red.tweenTo(Colors.blue), // <-- define tween of colors builder: (context, child, value) { // <-- builder function return Container( color: value, // <-- use animated value width: 100, height: 100 ); });
2.) MultiTween
enum AniProps { width, height, color } // <-- define properties class MyWidget extends StatelessWidget { final _tween = MultiTween() // <-- design tween ..add(AniProps.width, 0.0.tweenTo(400.0), 1000.milliseconds) ..add(AniProps.width, 400.0.tweenTo(300.0), 1000.milliseconds) ..add(AniProps.height, 500.0.tweenTo(200.0), 2000.milliseconds) ..add(AniProps.color, Colors.red.tweenTo(Colors.blue), 2.seconds); @override Widget build(BuildContext context) { return ... // <-- use tween } }
3.) Anicoto
class _MyAnimatedWidgetState extends Statewith AnimationMixin { // <-- add AnimationMixin to state class Animation size; // <-- declare animation variable @override void initState() { size = 0.0.tweenTo(200.0).animatedBy(controller); // <-- connect tween and controller and apply to animation variable controller.play(); // <-- start the animation playback super.initState(); } @override Widget build(BuildContext context) { return Container( width: size.value, // <-- use animation variable's value here height: size.value, // <-- use animation variable's value here color: Colors.red ); } }
Staggered Animations
This is used for making ListView items to display with some animations. We can also perform these animations in GridView, Column and Row.
Add the following dependency inside the “pubspec.yaml”.
dependencies:
flutter_staggered_animations: "^0.1.2"
Add the following package to get all the features present in Staggered Animations.
import 'package:flutter_staggered_animations/flutter_staggered_animations.dart';
Basic Use
Basic usage of Staggered Animations which we can use in the ListView.
@override Widget build(BuildContext context) { return Scaffold( body: AnimationLimiter( child: ListView.builder( itemCount: 100, itemBuilder: (BuildContext context, int index) { return AnimationConfiguration.staggeredList( position: index, duration: const Duration(milliseconds: 375), child: SlideAnimation( verticalOffset: 50.0, child: FadeInAnimation( child: YourListChild(), ), ), ); }, ), ), ); }
This package contains three types of classes for making staggered animations.
1.) Animation
2.) AnimationConfiguration
3.) AnimationLimiter
We can use different types of animations to make items of list to display accordingly.
- FadeInAnimation
- SlideAnimation
- ScaleAnimation
- FlipAnimation
Folding Cell
It is a simple folding widget where we can hide our main content and show it when clicked on open. It is available on both iOS & Android and also on the Web.
Add the following dependency into your “pubspec.yaml” file in order to access all the features in it.
dependencies:
folding_cell: "^1.0.0"
Add the following package
import 'package:folding_cell/folding_cell.dart';
Basic Usage
We need to create all the cards to show the information to the user.
v 1.0.0
class FoldingCellSimpleDemo extends StatelessWidget { final _foldingCellKey = GlobalKey(); @override Widget build(BuildContext context) { return Container( color: Color(0xFF2e282a), alignment: Alignment.topCenter, child: SimpleFoldingCell.create( key: _foldingCellKey, frontWidget: _buildFrontWidget(), innerWidget: _buildInnerWidget(), cellSize: Size(MediaQuery.of(context).size.width, 140), padding: EdgeInsets.all(15), animationDuration: Duration(milliseconds: 300), borderRadius: 10, onOpen: () => print('cell opened'), onClose: () => print('cell closed'), ), ); } Widget _buildFrontWidget() { return Container( color: Color(0xFFffcd3c), alignment: Alignment.center, child: Stack( children: [ Align( alignment: Alignment.center, child: Text( "CARD TITLE", style: GoogleFonts.aldrich( color: Color(0xFF2e282a), fontSize: 20.0, fontWeight: FontWeight.bold, ), ), ), Positioned( right: 5, bottom: 0, child: FlatButton( onPressed: () => _foldingCellKey?.currentState?.toggleFold(), child: Text( "OPEN", ), textColor: Colors.white, color: Colors.indigoAccent, splashColor: Colors.white.withOpacity(0.5), ), ) ], ), ); } Widget _buildInnerWidget() { return Container( color: Color(0xFFecf2f9), padding: EdgeInsets.only(top: 10), child: Stack( children: [ Align( alignment: Alignment.topCenter, child: Text( "CARD TITLE", style: GoogleFonts.aldrich( color: Color(0xFF2e282a), fontSize: 22.0, fontWeight: FontWeight.bold, ), ), ), Align( alignment: Alignment.center, child: Text( "CARD DETAIL", style: GoogleFonts.aldrich( color: Color(0xFF2e282a), fontSize: 40.0, ), ), ), Positioned( right: 5, bottom: 0, child: FlatButton( onPressed: () => _foldingCellKey?.currentState?.toggleFold(), child: Text( "Close", ), textColor: Colors.white, color: Colors.indigoAccent, splashColor: Colors.white.withOpacity(0.5), ), ), ], ), ); } }
v 0.1.2
class FoldingCellSimpleDemo extends StatelessWidget { final _foldingCellKey = GlobalKey(); @override Widget build(BuildContext context) { return Container( color: Color(0xFF2e282a), alignment: Alignment.topCenter, child: SimpleFoldingCell( key: _foldingCellKey, frontWidget: _buildFrontWidget(), innerTopWidget: _buildInnerTopWidget(), innerBottomWidget: _buildInnerBottomWidget(), cellSize: Size(MediaQuery.of(context).size.width, 125), padding: EdgeInsets.all(15), animationDuration: Duration(milliseconds: 300), borderRadius: 10, onOpen: () => print('cell opened'), onClose: () => print('cell closed')), ); } Widget _buildFrontWidget() { return Container( color: Color(0xFFffcd3c), alignment: Alignment.center, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text("CARD", style: TextStyle( color: Color(0xFF2e282a), fontFamily: 'OpenSans', fontSize: 20.0, fontWeight: FontWeight.w800)), FlatButton( onPressed: () => _foldingCellKey?.currentState?.toggleFold(), child: Text( "Open", ), textColor: Colors.white, color: Colors.indigoAccent, splashColor: Colors.white.withOpacity(0.5), ) ], )); } Widget _buildInnerTopWidget() { return Container( color: Color(0xFFff9234), alignment: Alignment.center, child: Text("TITLE", style: TextStyle( color: Color(0xFF2e282a), fontFamily: 'OpenSans', fontSize: 20.0, fontWeight: FontWeight.w800))); } Widget _buildInnerBottomWidget() { return Container( color: Color(0xFFecf2f9), alignment: Alignment.bottomCenter, child: Padding( padding: EdgeInsets.only(bottom: 10), child: FlatButton( onPressed: () => _foldingCellKey?.currentState?.toggleFold(), child: Text( "Close", ), textColor: Colors.white, color: Colors.indigoAccent, splashColor: Colors.white.withOpacity(0.5), ), ), ); } }