What is AEC 256 Encryption & Android AEC Encryption
You may have heard of advanced encryption standard, or AES for short but may not know the answer to the question “what is AES 256?” Here are four things you need to know about AES:
Advanced Encryption Standard (AES) is a widely-used encryption algorithm that is used to secure sensitive data, such as financial information and passwords. It is a symmetric-key algorithm, which means that the same key is used for both encrypting and decrypting the data.
AES was developed by the National Institute of Standards and Technology (NIST) and is considered to be a very secure encryption method. It uses a fixed block size of 128 bits and a key size of 128, 192, or 256 bits. The longer the key size, the stronger the encryption. For example, AES256 uses a 256-bit key, which is considered to be very secure.
AES is often used in conjunction with other encryption technologies, such as Transport Layer Security (TLS) and Secure Sockets Layer (SSL), to secure data transmission over the internet. It is also used in a variety of other applications, such as disk encryption and secure shell (SSH) connections.
- What’s behind the name? AES is a variant of the Rijndael family of symmetric block cipher algorithms, which was developed by Belgian cryptographers Joan Daemen and Vincent Rijmen. The Rijndael algorithm was chosen by the National Institute of Standards and Technology (NIST) to be the new Advanced Encryption Standard (AES), replacing the previously used Data Encryption Standard (DES). AES is a symmetric-key algorithm that uses a fixed block size of 128 bits and a key size of 128, 192, or 256 bits. The key size determines the strength of the encryption, with longer key sizes offering stronger security. AES256 uses a 256-bit key, which is considered to be very secure and is widely used to protect sensitive data. The name "Rijndael" is a combination of the cryptographers' last names, with "Rij" coming from Rijmen and "ndael" coming from Daemen. The name "AES" is simply the acronym for the Advanced Encryption Standard.
- Where is AES used?Android AES 256 encryption/decrypt example is commonly used in a lot of ways, including wireless security, processor security, file encryption, and SSL/TLS. In fact, your web browser probably used AES to encrypt your connection with this website.
- Why is AES so popular? AES is popular because it is fast, secure, and widely available. It was adopted as the standard encryption algorithm by the US government in 2002, and it is now used by millions of individuals and organizations around the world. One of the main reasons for its popularity is that it has been thoroughly analyzed and tested by security experts, and no significant vulnerabilities have been found to date. It is also relatively fast to execute, making it practical for use in a wide range of applications. Additionally, AES is available in many software libraries and hardware implementations, making it easy to use in a variety of systems.
- How secure is AES? With a 256-bit encryption key, AES is very secure — virtually unbreakable. (More on that in just minute.)
What is the best algorithm for encryption?
The Advanced Encryption Standard (AES) is the trusted standard algorithm used by the United States government, as well as other organizations. Although extremely efficient in the 128-bit form, AES also uses 192- and 256-bit keys for very demanding encryption purposes.Dec
Advanced Encryption Standard is built from three block ciphers :
- AES-128
- AES-192
- AES-256
All symmetric encryption ciphers use the same key for encryption and decryption. Which means in the sense, both the sender and receiver must have the same key. Each encrypts and decrypts have 128 bits data in chunks by using cryptographic keys 128-, 192- or 256- bits.
Plain text which we use to convert are first converted into Cipher and then outputs into Cipher text bits of 128, 192 & 256.
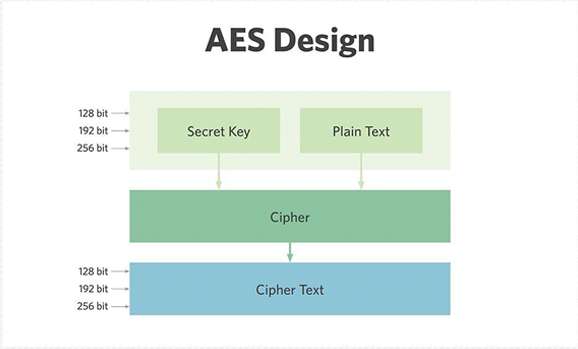
Example of AES 256 Encrypt/Decrypt in Android with Demo
We need to initialize KeyGenerator and SecretKey in order to make our own Key.
KeyGenerator keyGenerator;
SecretKey secretKey;
Prevent a parameter with getInstance method and initialize the KeyGenerator with keysize 256. Add them in the try catch method to prevent crashes.
try { keyGenerator = KeyGenerator.getInstance("AES"); keyGenerator.init(256); secretKey = keyGenerator.generateKey(); } catch (Exception e) { e.printStackTrace(); }
Now I’m using IV(Initialization Vector) in my code. This is an optional part in AES Encryption but a way better to use. Use it with SecureRandom class.
byte[] IV = new byte[16]; SecureRandom random;
Initialize the IV after declaring it globally.
random = new SecureRandom(); random.nextBytes(IV);
Encryption Method:
public static byte[] encrypt(byte[] plaintext, SecretKey key, byte[] IV) throws Exception { Cipher cipher = Cipher.getInstance("AES"); SecretKeySpec keySpec = new SecretKeySpec(key.getEncoded(), "AES"); IvParameterSpec ivSpec = new IvParameterSpec(IV); cipher.init(Cipher.ENCRYPT_MODE, keySpec, ivSpec); byte[] cipherText = cipher.doFinal(plaintext); return cipherText; }
Decryption Method:
public static String decrypt(byte[] cipherText, SecretKey key, byte[] IV) { try { Cipher cipher = Cipher.getInstance("AES"); SecretKeySpec keySpec = new SecretKeySpec(key.getEncoded(), "AES"); IvParameterSpec ivSpec = new IvParameterSpec(IV); cipher.init(Cipher.DECRYPT_MODE, keySpec, ivSpec); byte[] decryptedText = cipher.doFinal(cipherText); return new String(decryptedText); } catch (Exception e) { e.printStackTrace(); } return null; }
How to call both the methods:
I’ve used an EditText for the user text field to type whatever the string which needs to be encrypted. Two buttons for getting results respectively. One for Encrypting and other for Decrypting. Also used two TextViews for displaying the values(results). One text is used for displaying encrypted value and the other one for decrypted value.
btn1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { try { encrypt = encrypt(et.getText().toString().getBytes(), secretKey, IV); String encryptText = new String(encrypt, "UTF-8"); tv1.setText(encryptText); } catch (Exception e) { e.printStackTrace(); } } }); btn2.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { try { String decrypt = decrypt(encrypt, secretKey, IV); tv2.setText(decrypt); } catch (Exception e) { e.printStackTrace(); } } });
After placing these all you can now see the results of how Encrypt and Decrypt are done through AES 256 Encryption.
Remember that IV(Initialization Vector) is an arbitrary number that is only used along with a secret key for data encryption. To generate the IV we use the SecureRandom class.
From the Initialization vector, We create an IvParameterSpec which is always required when creating the cipher during encryption or decryption.
You can see the output in the below example:
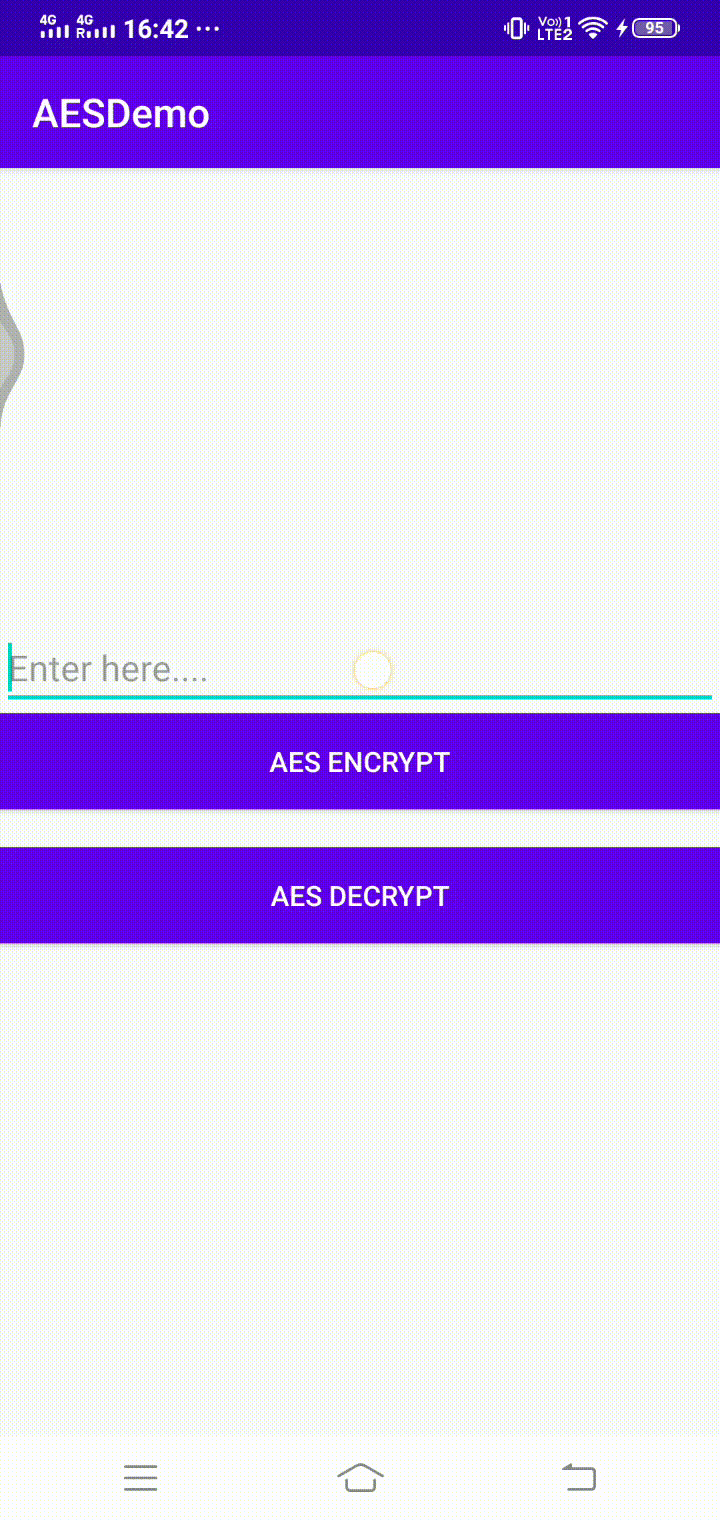
Connect with our team of Amar InfoTech Android Security experts for assistance with implementing AES 256 encryption in your android project. Our team has experience in implementing AES encryption android projects and can provide the necessary support to ensure a successful implementation.