Integrating Facebook is the easiest/simplest way in Android devices. Rather than custom login/registration we can do Facebook login in the android app using the current Facebook user account. We can authenticate people with their Facebook credentials. We can also enable the sharing of content through Facebook integration. We need to integrate the Facebook API in order to get all the user credentials from Facebook.
Before we deep dive into code, we should get facebook ID from facebook console. You can go through that URL and login with your current Facebook account. Create a project if not created by anyone. You need to redirect through “settings->Basic” on the side menu where you can find your Facebook ID. But before copying the ID we need to make sure that our project is added there. By scrolling down you can see the “Add Platform” label, clicking on it you’ll be given information on how to add the package name(Current project in android) and key hashes.
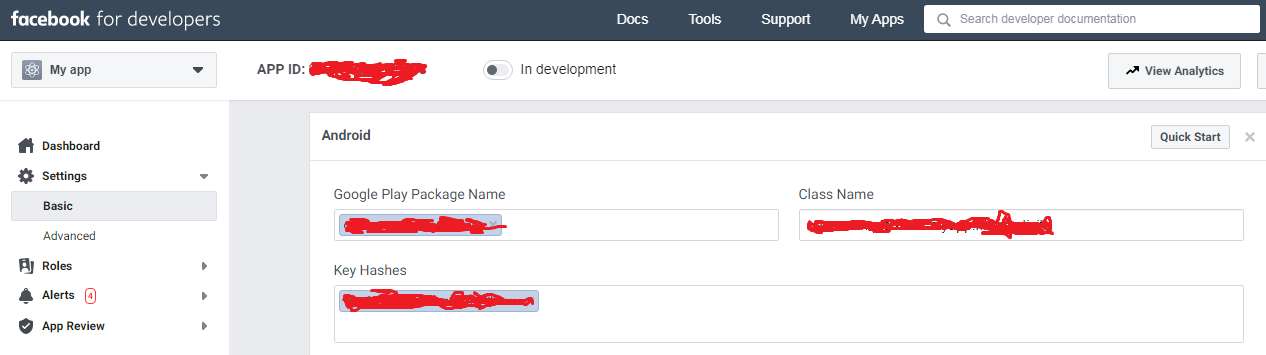
As you can see in the above picture I’ve created my project and add package name and key hashes. You can get the package name from the android studio in the manifest/Gradle file. Now the question is how do we get key hashes?
How to generate key hash?
We can either generate by using some of the commands present in the getting started Facebook for android SDK. The second way of generating key hash is by using code. You can use the below code on your First activity launch in onCreate method to print the generated key hash.
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Add code to print out the key hash try { PackageInfo info = getPackageManager().getPackageInfo( "com.facebook.samples.hellofacebook", PackageManager.GET_SIGNATURES); for (Signature signature : info.signatures) { MessageDigest md = MessageDigest.getInstance("SHA"); md.update(signature.toByteArray()); Log.d("KeyHash:", Base64.encodeToString(md.digest(), Base64.DEFAULT)); } } catch (NameNotFoundException e) { } catch (NoSuchAlgorithmException e) { } ...
Note: This code will only generate debug SHA key, If you want to build a release SHA key then you need to follow the link which I’ve posted above. Using commands you can build release key hashes.

In your logcat in android studio, you can see the key hashes printed somewhat like this shown on the above picture.
Time to get into the code
In order to use the Facebook SDK for android application, you need to add some of the dependencies.
In your Gradle Scripts | build.Gradle (Project:
mavenCentral()
In your Gradle Scripts | build. Gradle (Module: app) module add the following dependency.
implementation 'com.facebook.android:facebook-android-sdk:[5,6)'
Save and Sync your project once in order to get all the functionalities used in facebook login SDK.
Now open your Strings xml file which is located under /app/res/values/strings.xml and add the following code
222005321890848
Replace your facebook ID which we generated recently in Facebook Console.
Make sure to add INTERNET permission inside the Manifest file.
uses-permission android:name="android.permission.INTERNET"
Stay remain inside the manifest file because now we need to add the facebook activity inside here.
meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id" activity android:name="com.facebook.FacebookActivity" android:configChanges= "keyboard|keyboardHidden|screenLayout|screenSize|orientation" android:label="@string/app_name" activity android:name="com.facebook.CustomTabActivity" android:exported="true" intent-filter action android:name="android.intent.action.VIEW" category android:name="android.intent.category.DEFAULT" category android:name="android.intent.category.BROWSABLE" data android:scheme="@string/fb_login_protocol_scheme" intent-filter activity
It is used for opening up the facebook activity and also the use of intent filters for Chrome Custom Tabs.
Note - make sure you add these code lines inside the
Designing facebook button
Go to the xml file where you want to use the facebook integration and paste the following code. Make sure you design the facebook button according to yourself.
We can also use the custom button if not the default one.
Register the callback class
callbackManager = CallbackManager.Factory.create();
Initialize the callback manager inside the onCreate method. This handles the login responses. If you are adding this in fragment then add it under onCreateView() method.
We need to set up some permissions in order to access the details from facebook.
Make sure if you are using the default facebook button then you are required to initialize with default button, other than you have to initialize facebook SDK with LoginManager if you are not using the default button.
Add the permissions whichever we needed them on call back listener. It sends you the result on what your requirements are.
Also we need to handle the onActivityResult method in order to read the result back from facebook SDK.
With default button
private static final String EMAIL = "email"; loginButton = (LoginButton) findViewById(R.id.login_button); loginButton.setReadPermissions(Arrays.asList(EMAIL)); // If you are using in a fragment, call loginButton.setFragment(this); // Callback registration loginButton.registerCallback(callbackManager, new FacebookCallback() { @Override public void onSuccess(LoginResult loginResult) { // App code } @Override public void onCancel() { // App code } @Override public void onError(FacebookException exception) { // App code } });
With custom button
callbackManager = CallbackManager.Factory.create(); LoginManager.getInstance().registerCallback(callbackManager, new FacebookCallback() { @Override public void onSuccess(LoginResult loginResult) { // App code } @Override public void onCancel() { // App code } @Override public void onError(FacebookException exception) { // App code } });
Make sure you add the call back class inside the onActivityResult
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { callbackManager.onActivityResult(requestCode, resultCode, data); super.onActivityResult(requestCode, resultCode, data); }
Tips to Hire a Mobile app development Company that can grab your vision.
Develop your first android mobile application using facebook login integration with Amar Infotech. In case any of your questions remain unanswered, you can directly talk to us, or leave us an email at sales@amarinfotech.com.